9-Day Machine Learning Mastery
9-Day Machine Learning Mastery
Course Overview
Day 1: Introduction to Machine Learning
- Theory & Notes:
- What is ML? Types: Supervised, Unsupervised, Reinforcement Learning.
- Overview of common ML algorithms.
- Bias-Variance tradeoff, underfitting vs. overfitting.
- Evaluation metrics: Accuracy, Precision, Recall, F1-score, ROC-AUC.
- Technical Stuff:
- Install Python, Jupyter Notebook, NumPy, Pandas, Matplotlib, and Scikit-Learn.
- Hands-on Project:
- Load a dataset (e.g., Titanic dataset).
- Perform exploratory data analysis (EDA): missing values, distributions, correlations.
Day 2: Data Preprocessing & Feature Engineering
- Theory & Notes:
- Data cleaning, handling missing values, encoding categorical variables.
- Feature scaling: MinMaxScaler, StandardScaler.
- Feature selection techniques.
- Technical Stuff:
- Using
Pandas
&Scikit-Learn
for preprocessing.
- Using
- Hands-on Project:
- Work with the Titanic dataset: clean data, handle missing values, and engineer new features.
Day 3: Supervised Learning - Regression Models
- Theory & Notes:
- Linear Regression, Polynomial Regression.
- Ridge, Lasso Regression.
- Gradient Descent, Cost Function.
- Technical Stuff:
- Implement Linear Regression from scratch using NumPy.
- Use
Scikit-Learn
for regression models.
- Hands-on Project:
- Predict house prices using the Boston Housing dataset.
Day 4: Supervised Learning - Classification Models
- Theory & Notes:
- Logistic Regression, Decision Trees, Random Forest, Support Vector Machines (SVM).
- Hyperparameter tuning (GridSearchCV, RandomizedSearchCV).
- Technical Stuff:
- Implement a decision tree and logistic regression classifier using
Scikit-Learn
.
- Implement a decision tree and logistic regression classifier using
- Hands-on Project:
- Classify whether a person has diabetes using the Pima Indians Diabetes dataset.
Day 5: Unsupervised Learning - Clustering & Dimensionality Reduction
- Theory & Notes:
- K-Means, Hierarchical Clustering, DBSCAN.
- PCA (Principal Component Analysis), t-SNE.
- Technical Stuff:
- Implement K-Means and PCA using
Scikit-Learn
.
- Implement K-Means and PCA using
- Hands-on Project:
- Customer segmentation using the Mall Customers dataset.
Day 6: Neural Networks & Deep Learning (Basics)
- Theory & Notes:
- Introduction to Artificial Neural Networks (ANN).
- Activation functions (ReLU, Sigmoid, Softmax).
- Backpropagation and optimization.
- Technical Stuff:
- Use
TensorFlow
andKeras
to build a simple neural network.
- Use
- Hands-on Project:
- Classify handwritten digits using the MNIST dataset.
Day 7: Advanced Deep Learning - CNNs & RNNs
- Theory & Notes:
- Convolutional Neural Networks (CNNs) and Recurrent Neural Networks (RNNs).
- Long Short-Term Memory (LSTM) networks.
- Technical Stuff:
- Build CNNs and LSTMs using
TensorFlow/Keras
.
- Build CNNs and LSTMs using
- Hands-on Project:
- Build an image classifier using the CIFAR-10 dataset.
- Sentiment analysis on movie reviews using LSTMs.
Day 8: Reinforcement Learning & Model Deployment
- Theory & Notes:
- Introduction to Reinforcement Learning, Q-Learning.
- Model deployment using Flask/FastAPI.
- Technical Stuff:
- Use OpenAI Gym for reinforcement learning.
- Deploy a trained model using Flask.
- Hands-on Project:
- Train an agent to play CartPole using OpenAI Gym.
Day 9: Real-World Project & Final Review
- Final Project:
- Pick a real-world dataset (Kaggle, UCI ML Repository).
- Apply everything learned (EDA, feature engineering, model training, evaluation).
- Deploy the model and document results.
- Final Review:
- Revise notes, revisit concepts, and ensure a strong understanding.
Let's start with Day 1! 🚀
Day 1: Introduction to Machine Learning - Detailed Plan
1. Theory & Notes
Before diving into coding, start by understanding the core concepts of machine learning.
What is Machine Learning?
Machine learning is a field of artificial intelligence where computers learn from data to make predictions or decisions without being explicitly programmed.
Types of Machine Learning
- Supervised Learning: Model learns from labeled data (input-output pairs).
- Example: Predicting house prices based on past sales data.
- Unsupervised Learning: Model finds patterns in unlabeled data.
- Example: Clustering customers based on shopping behavior.
- Reinforcement Learning: Model learns through rewards and penalties.
- Example: Training an AI to play chess.
Common ML Algorithms
- Supervised Learning:
- Regression: Linear Regression, Decision Trees, Random Forests
- Classification: Logistic Regression, SVM, Neural Networks
- Unsupervised Learning:
- Clustering: K-Means, DBSCAN
- Dimensionality Reduction: PCA
- Reinforcement Learning:
- Q-Learning, Deep Q Networks (DQN)
Bias-Variance Tradeoff
- Bias: Model makes strong assumptions, leading to underfitting.
- Variance: Model is too complex and captures noise, leading to overfitting.
- Goal: Find a balance between the two.
Evaluation Metrics for Supervised Learning
-
Classification:
- Accuracy = Correct Predictions / Total Predictions
- Precision = TP / (TP + FP) → How many selected items are relevant?
- Recall = TP / (TP + FN) → How many relevant items are selected?
- F1-Score = 2 × (Precision × Recall) / (Precision + Recall)
- ROC-AUC (Receiver Operating Characteristic - Area Under Curve): Measures the performance across thresholds.
-
Regression:
- Mean Squared Error (MSE)
- Mean Absolute Error (MAE)
- R² Score (Coefficient of Determination)
2. Technical Stuff - Setting Up Environment
Make sure you have the required libraries installed.
Install Python & Essential Libraries
pip install numpy pandas matplotlib seaborn scikit-learn jupyter
Start Jupyter Notebook
jupyter notebook
Once the Jupyter Notebook opens, you can start coding in interactive cells.
3. Hands-on Project: Titanic Dataset EDA
Dataset: Titanic Survival Prediction
- Dataset Source: Kaggle Titanic Dataset
- Goal: Perform Exploratory Data Analysis (EDA) to understand the data.
- Tasks:
- Load the dataset.
- Explore missing values.
- Visualize distributions.
- Find correlations.
Code Template: Titanic EDA
# Importing Libraries
import numpy as np
import pandas as pd
import matplotlib.pyplot as plt
import seaborn as sns
# Load Dataset
titanic = pd.read_csv("https://raw.githubusercontent.com/datasciencedojo/datasets/master/titanic.csv")
# Display first 5 rows
titanic.head()
Step 1: Check Missing Values
# Check for missing values
titanic.isnull().sum()
Step 2: Handle Missing Values
# Fill missing Age with median
titanic['Age'].fillna(titanic['Age'].median(), inplace=True)
# Fill missing Embarked with most frequent value
titanic['Embarked'].fillna(titanic['Embarked'].mode()[0], inplace=True)
# Drop Cabin column due to high missing values
titanic.drop(columns=['Cabin'], inplace=True)
Step 3: Data Distribution
# Visualizing Age distribution
sns.histplot(titanic['Age'], bins=30, kde=True)
plt.title('Age Distribution')
plt.show()
Step 4: Correlation Heatmap
# Check correlation between numerical features
plt.figure(figsize=(8,6))
sns.heatmap(titanic.corr(), annot=True, cmap="coolwarm")
plt.title('Feature Correlation')
plt.show()
Step 5: Survival Rate by Gender
# Countplot of Survival based on Gender
sns.countplot(x='Survived', hue='Sex', data=titanic)
plt.title('Survival Rate by Gender')
plt.show()
Step 6: Conclusion from EDA
- Missing values were handled.
- Older people had a different survival rate than younger passengers.
- Women had a higher survival rate than men.
Concepts to Learn for Other Days
To strengthen your foundation, make sure to learn:
- Probability & Statistics: Important for understanding algorithms.
- Linear Algebra: Needed for deep learning (e.g., matrices, vectors).
- Gradient Descent: Core optimization technique in ML.
- Overfitting & Regularization: L1/L2 regularization, dropout.
- Feature Engineering: Handling categorical & numerical features effectively.
This foundation will help you understand supervised learning, deep learning, and advanced techniques in the coming days.
Would you like me to modify anything or add more practical exercises? 🚀
Add a Comment
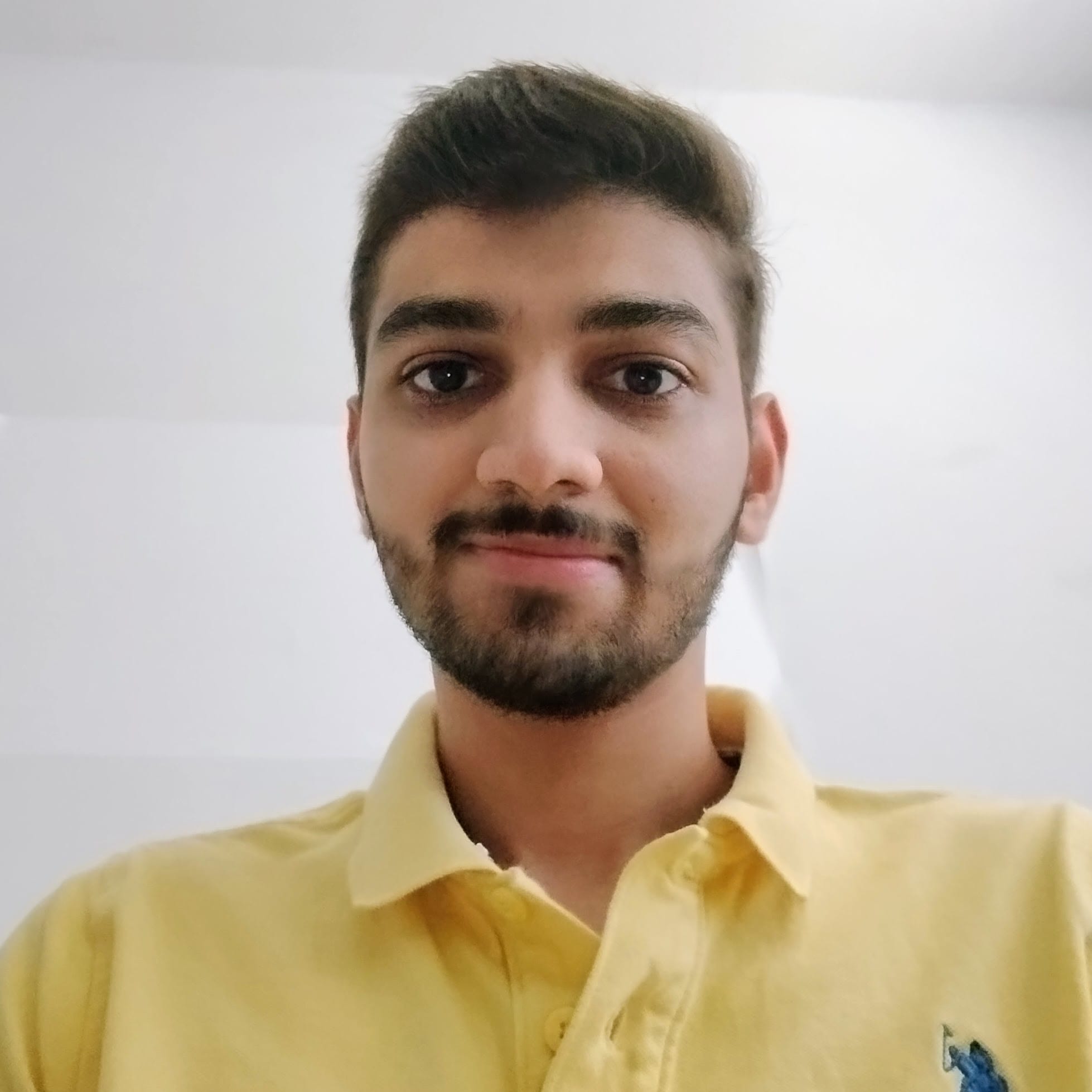
Archit Rathod
Software Developer and tech enthusiast. Passionate about web development and creating user-friendly experiences.