React.js Crash Course
React.js Crash Course
Module 1: Introduction to React:
What is React.js?
React.js, commonly known as React, is an open-source JavaScript library for building user interfaces or UI components. It was developed by Facebook and is maintained by Facebook and a community of individual developers and companies. React is widely used for creating single-page applications where data can change over time without reloading the page. It allows developers to build reusable UI components and manage the state of an application efficiently.
Why use React.js?
- Declarative Syntax: React uses a declarative syntax, making it easier to understand and debug.
- Component-Based Architecture: React follows a component-based architecture, enabling the creation of reusable and modular UI components.
- Virtual DOM: React employs a virtual DOM, which enhances performance by minimizing direct manipulation of the actual DOM.
- One-Way Data Binding: React follows a unidirectional data flow, simplifying the tracking of data changes.
- Large Ecosystem: React has a vast ecosystem, including tools and libraries, contributing to its popularity.
Virtual DOM Concept:
The Virtual DOM is a programming concept where an ideal, or "virtual," representation of the user interface is kept in memory. It acts as a lightweight copy of the real DOM and is used for efficient updates. When the state of an object changes, React first updates the Virtual DOM, then compares it to the current state, and finally updates only the parts of the actual DOM that have changed. This minimizes the number of manipulations needed, improving performance.
JSX Syntax:
JSX (JavaScript XML) is a syntax extension for JavaScript recommended by React. It looks similar to XML/HTML but ultimately gets transpiled to JavaScript. JSX allows developers to write UI components in a syntax that closely resembles HTML, making the code more readable.
Example of JSX:
const element = <h1>Hello, React!</h1>;
In the above code, <h1>Hello, React!</h1>
is JSX, representing a React element. This JSX will be transformed into a React.createElement
function call during the compilation process.
Output:
<h1>Hello, React!</h1>
Understanding these foundational concepts is crucial for diving into React development and building efficient and scalable applications.
Module 2: Setting Up Your Development Environment:
Node.js and npm Installation:
-
Node.js: Node.js is a JavaScript runtime that allows you to run JavaScript on the server side. React development often relies on Node.js for package management and running scripts.
-
npm (Node Package Manager): npm is the default package manager for Node.js. It is used to install, share, and manage dependencies. React projects typically leverage npm to manage libraries and tools.
Installation:
- Download and install Node.js from nodejs.org.
- npm is included with Node.js, so there is no need to install it separately.
Create React App:
-
Create React App (CRA): CRA is a command-line tool that sets up a new React project with a sensible default configuration. It allows developers to quickly start building React applications without dealing with complex configurations.
Create a New React App:
npx create-react-app my-react-app cd my-react-app
This command creates a new React app named
my-react-app
and navigates into its directory.Start the Development Server:
npm start
This command starts the development server, and you can view your React app at
http://localhost:3000
in your web browser.
Understanding the Project Structure:
A typical Create React App project structure looks like this:
my-react-app/
│
├── public/
│ ├── index.html
│ └── favicon.ico
│
├── src/
│ ├── index.js
│ ├── App.js
│ ├── App.css
│ ├── index.css
│ └── logo.svg
│
├── package.json
├── README.md
└── ...
-
public/
: Contains static assets such as HTML files, images, and the favicon. -
src/
: Houses the source code for the React application.index.js
: Entry point of the application where React is rendered into the DOM.App.js
: The main component that gets rendered inindex.js
.App.css
andindex.css
: Style files for the components.logo.svg
: An example image.
-
package.json
: Configuration file that includes project metadata and dependencies. -
README.md
: Documentation for the project.
Understanding the project structure is essential for organizing code and assets effectively during React development. The entry point, src/index.js
, is where you'll find the initial setup and rendering of the React application.
Module 3: Components and Props:
Functional Components:
-
Functional Component Definition: A functional component is a JavaScript function that takes
props
(short for properties) as an argument and returns React elements. It is a simple way to define stateless or presentational components.Example:
// Functional Component const Greeting = (props) => { return <h1>Hello, {props.name}!</h1>; };
Usage:
<Greeting name="John" />
Class Components:
-
Class Component Definition: Class components are ES6 classes that extend
React.Component
. They have arender
method and can have state and lifecycle methods.Example:
// Class Component class Welcome extends React.Component { render() { return <h1>Welcome, {this.props.user}!</h1>; } }
Usage:
<Welcome user="Alice" />
Props and PropTypes:
-
Props in Functional Components: Props are passed as arguments to functional components and accessed as properties of the
props
object.Example:
const Person = (props) => { return ( <p> Name: {props.name}, Age: {props.age} </p> ); };
Usage:
<Person name="Bob" age={30} />
-
PropTypes for Type Checking: PropTypes is a library for type-checking props during development. It helps catch bugs related to incorrect prop types.
Example:
import PropTypes from "prop-types"; const Book = (props) => { return <h2>Title: {props.title}</h2>; }; Book.propTypes = { title: PropTypes.string.isRequired, };
State and setState:
-
State in Class Components: State is used for managing component-specific data that may change over time. Class components can have state.
Example:
class Counter extends React.Component { constructor(props) { super(props); this.state = { count: 0 }; } render() { return ( <div> <p>Count: {this.state.count}</p> </div> ); } }
-
setState for Updating State:
setState
is used to update the state of a component. It takes an object that represents the updated state or a function that returns the updated state.Example:
class Counter extends React.Component { constructor(props) { super(props); this.state = { count: 0 }; } handleClick = () => { this.setState({ count: this.state.count + 1 }); }; render() { return ( <div> <p>Count: {this.state.count}</p> <button onClick={this.handleClick}>Increment</button> </div> ); } }
Understanding functional components, class components, props, and state is fundamental to React development. Functional components are simpler and recommended for presentational components, while class components offer additional features like state and lifecycle methods. Props enable the passing of data between components, and state allows components to manage their internal data. The setState
method is crucial for updating the state and triggering re-renders.
Module 4: JSX (JavaScript XML):
Understanding JSX Syntax:
-
JSX Definition: JSX, or JavaScript XML, is a syntax extension for JavaScript. It allows you to write HTML-like code in your JavaScript files, making it easier to describe what the UI should look like.
Example:
const element = <h1>Hello, JSX!</h1>;
Output:
<h1>Hello, JSX!</h1>
In this example, the JSX
<h1>Hello, JSX!</h1>
gets transpiled into aReact.createElement
function call during the compilation process.
JSX Expressions:
-
Embedding Expressions in JSX: You can embed JavaScript expressions within curly braces
{}
in JSX. This allows you to dynamically include values or execute functions.Example:
const name = "John"; const element = <p>Hello, {name}!</p>;
Output:
<p>Hello, John!</p>
Here, the value of the
name
variable is dynamically inserted into the JSX.
Conditional Rendering in JSX:
-
Using Ternary Operator: You can use the ternary operator to conditionally render elements based on a condition.
Example:
const isLoggedIn = true; const greeting = isLoggedIn ? <p>Welcome back!</p> : <p>Please log in</p>;
Output:
<p>Welcome back!</p>
-
Using Logical && Operator: The logical
&&
operator can be used for concise conditional rendering. The right-hand expression is rendered only if the left-hand expression is truthy.Example:
const hasMessages = true; const messages = hasMessages && <p>You have new messages</p>;
Output:
<p>You have new messages</p>
-
Using Conditional Statements: You can also use traditional
if
statements outside of JSX to conditionally render different parts of your UI.Example:
function Greeting({ isLoggedIn }) { if (isLoggedIn) { return <p>Welcome back!</p>; } else { return <p>Please log in</p>; } }
Usage:
<Greeting isLoggedIn={true} />
Understanding JSX syntax is crucial for developing React applications. JSX provides a more concise and readable way to describe the structure of UI components. Embedding expressions allows dynamic content, and conditional rendering is a common pattern in React applications for showing different content based on conditions.
Module 5: Handling Events:
Event Handling in React:
-
Event Syntax: Handling events in React is similar to handling events in HTML. You use camelCase naming convention for event names, and you assign a function as the event handler.
Example:
function handleClick() { console.log("Button Clicked"); } const buttonElement = <button onClick={handleClick}>Click me</button>;
Clicking the button will log 'Button Clicked' to the console.
Binding Event Handlers:
-
Binding in Class Components: When using class components, you need to bind the event handler function to the class instance in the constructor, ensuring that
this
refers to the component instance.Example:
class ButtonClick extends React.Component { constructor(props) { super(props); this.handleClick = this.handleClick.bind(this); } handleClick() { console.log("Button Clicked"); } render() { return <button onClick={this.handleClick}>Click me</button>; } }
-
Binding with Arrow Function: Alternatively, you can use an arrow function in the event handler directly. This way, the function inherits the
this
value from the enclosing scope.Example:
class ButtonClick extends React.Component { handleClick = () => { console.log("Button Clicked"); }; render() { return <button onClick={this.handleClick}>Click me</button>; } }
Using Arrow Functions for Event Handlers:
-
Arrow Functions for Inline Event Handlers: When using functional components, you can use arrow functions directly in the event handler to avoid binding issues. This is often used for inline event handlers.
Example:
const FunctionalComponent = () => { const handleClick = () => { console.log("Button Clicked"); }; return <button onClick={handleClick}>Click me</button>; };
In this example, the arrow function
handleClick
is used directly in theonClick
event.
Passing Parameters to Event Handlers:
-
Binding with Arrow Function in Render: If you need to pass parameters to an event handler, you can use an arrow function in the
render
method.Example:
class ParameterExample extends React.Component { handleClick = (param) => { console.log("Button Clicked with parameter:", param); }; render() { const paramValue = "Hello, React!"; return ( <button onClick={() => this.handleClick(paramValue)}> Click me with parameter </button> ); } }
Understanding event handling is crucial for creating interactive React applications. The syntax for handling events is similar to HTML, but differences arise when working with class components and handling the this
context. Arrow functions provide a concise way to handle events, especially in functional components or when passing parameters to event handlers.
Module 6: Lists and Keys:
Rendering Lists in React:
-
Mapping over Arrays: In React, you often render lists of elements by mapping over an array and creating React elements for each item.
Example:
const numbers = [1, 2, 3, 4, 5]; const ListComponent = () => { return ( <ul> {numbers.map((number) => ( <li key={number}>{number}</li> ))} </ul> ); };
The
map
function is used to create a list ofli
elements from thenumbers
array.
Keys and Their Importance:
-
Key Attribute in Lists: In React, the
key
attribute is a special attribute used to uniquely identify elements in a list. It helps React identify which items have changed, are added, or are removed.Example:
const ListComponent = () => { const users = [ { id: 1, name: "Alice" }, { id: 2, name: "Bob" }, { id: 3, name: "Charlie" }, ]; return ( <ul> {users.map((user) => ( <li key={user.id}>{user.name}</li> ))} </ul> ); };
Here, the
key
attribute is set to theid
property of each user for a unique identifier. -
Importance of Keys: Keys are crucial for React to efficiently update the UI. They help React recognize which items have changed, are added, or are removed. They should be unique among siblings in a list.
Dynamic Lists:
-
Dynamic List from State: You can dynamically render lists based on the state of your component.
Example:
class DynamicList extends React.Component { constructor(props) { super(props); this.state = { fruits: ["Apple", "Banana", "Orange"], }; } render() { return ( <ul> {this.state.fruits.map((fruit, index) => ( <li key={index}>{fruit}</li> ))} </ul> ); } }
Here, the list of fruits is stored in the component's state, and the
map
function is used to render the list dynamically.
Understanding how to render lists in React is crucial for building dynamic and scalable UIs. The map
function is commonly used to transform an array of data into a list of React elements. Keys play a vital role in helping React efficiently update the UI when the list changes. They should be unique and stable across renders.
Module 7: Forms in React:
Controlled Components:
-
Controlled Component Definition: In React, a controlled component is a form element whose value is controlled by React state. This means that the component's state is the single source of truth for the input value.
Example:
class ControlledForm extends React.Component { constructor(props) { super(props); this.state = { inputValue: "", }; } handleChange = (event) => { this.setState({ inputValue: event.target.value }); }; render() { return ( <form> <label> Name: <input type="text" value={this.state.inputValue} onChange={this.handleChange} /> </label> </form> ); } }
In this example, the
input
element is a controlled component, and its value is controlled by theinputValue
state.
Form Submission and Handling:
-
Handling Form Submission: In React, you can handle form submissions by attaching an event handler to the
onSubmit
event of the form.Example:
class FormSubmission extends React.Component { handleSubmit = (event) => { event.preventDefault(); // Process form data here }; render() { return ( <form onSubmit={this.handleSubmit}> {/* Form inputs */} <button type="submit">Submit</button> </form> ); } }
The
event.preventDefault()
prevents the default form submission behavior, allowing you to handle the submission manually.
Form Validation:
-
Basic Validation with State: You can perform basic form validation by maintaining state to track the validity of form fields.
Example:
class FormValidation extends React.Component { constructor(props) { super(props); this.state = { email: "", validEmail: false, }; } handleEmailChange = (event) => { const email = event.target.value; const isValidEmail = email.includes("@"); // Basic validation this.setState({ email, validEmail: isValidEmail }); }; render() { return ( <form> <label> Email: <input type="text" value={this.state.email} onChange={this.handleEmailChange} /> </label> {this.state.validEmail ? ( <p>Email is valid</p> ) : ( <p>Email is not valid</p> )} </form> ); } }
In this example, the validity of the email is checked, and the result is stored in the
validEmail
state.
Understanding controlled components is crucial for managing form state in React. By controlling the form elements through React state, you can easily manipulate and validate form data. Handling form submissions allows you to customize the behavior when a form is submitted, and form validation ensures that user input meets certain criteria before submission.
Module 8: Component Lifecycle:
Mounting, Updating, and Unmounting Phases:
-
Component Lifecycle Phases: React components go through three main phases: mounting, updating, and unmounting.
- Mounting: Occurs when an instance of a component is being created and inserted into the DOM.
- Updating: Occurs when a component is being re-rendered as a result of changes to either its props or state.
- Unmounting: Occurs when a component is being removed from the DOM.
Lifecycle Methods:
-
Mounting Lifecycle Methods:
constructor()
: Called when the component is initialized.render()
: Responsible for rendering the component's UI.componentDidMount()
: Invoked after the component is mounted to the DOM.
-
Updating Lifecycle Methods:
shouldComponentUpdate(nextProps, nextState)
: Called before rendering when new props or state are received. Returns a boolean to determine if the component should re-render.render()
: Re-renders the component.componentDidUpdate(prevProps, prevState)
: Invoked after the component updates.
-
Unmounting Lifecycle Method:
componentWillUnmount()
: Called just before the component is unmounted and destroyed.
Example:
class LifecycleExample extends React.Component { constructor(props) { super(props); console.log("Constructor"); } componentDidMount() { console.log("Component did mount"); } componentDidUpdate(prevProps, prevState) { console.log("Component did update"); } componentWillUnmount() { console.log("Component will unmount"); } render() { console.log("Render"); return <p>Component Lifecycle Example</p>; } }
Understanding these methods helps control the behavior of components at different stages of their lifecycle.
useEffect
Hook:
-
Introduction to
useEffect
: In functional components, theuseEffect
hook is used to perform side effects in your components. It combines the functionality of various lifecycle methods.Example:
import React, { useEffect } from "react"; const EffectExample = () => { useEffect(() => { console.log("Component did mount (equivalent)"); return () => { console.log("Component will unmount (equivalent)"); }; }, []); // Empty dependency array means it runs once (like componentDidMount) useEffect( () => { console.log("Component did update (equivalent)"); // Cleanup function (equivalent to componentWillUnmount) return () => { console.log("Component will unmount (equivalent)"); }; }, [ /* dependency array */ ] ); // Specify dependencies for re-run (like componentDidUpdate) return <p>Effect Example</p>; };
The
useEffect
hook is versatile and allows you to handle side effects in functional components. The cleanup function insideuseEffect
is equivalent to thecomponentWillUnmount
lifecycle method.
Understanding the component lifecycle is crucial for managing component behavior at different stages. Class components have explicit lifecycle methods, while functional components use the useEffect
hook for similar functionality. Each phase of the lifecycle provides an opportunity to perform specific tasks, such as initializing state, fetching data, or cleaning up resources.
Module 9: State Management:
Context API:
-
Introduction to Context API: The Context API is a React feature that allows you to pass data through the component tree without having to pass props manually at every level.
Example:
// Create a context const ThemeContext = React.createContext(); // Provide a value at the top of the component tree const App = () => { const theme = "light"; return ( <ThemeContext.Provider value={theme}> <Toolbar /> </ThemeContext.Provider> ); }; // Consume the context in a nested component const Toolbar = () => { return ( <div> <ThemedButton /> </div> ); }; const ThemedButton = () => { // Consume the context value const theme = useContext(ThemeContext); return <button style={{ background: theme }}>Themed Button</button>; };
In this example, the
ThemeContext.Provider
at the top of the tree provides the theme value to all components that consume the context, such asThemedButton
.
State Lifting:
-
What is State Lifting: State lifting is a pattern in React where the state is moved up to a common ancestor component that needs to share its state with its descendants.
Example:
const ParentComponent = () => { const [count, setCount] = useState(0); const increment = () => { setCount(count + 1); }; return ( <div> <ChildComponent count={count} increment={increment} /> </div> ); }; const ChildComponent = ({ count, increment }) => { return ( <div> <p>Count: {count}</p> <button onClick={increment}>Increment</button> </div> ); };
In this example, the
count
state and theincrement
function are defined in theParentComponent
and passed down as props toChildComponent
.
Redux (Introduction):
-
What is Redux: Redux is a state management library for JavaScript applications, commonly used with React. It provides a predictable state container and helps manage the state of the entire application in a single store.
Installation:
npm install redux react-redux
Example:
// Redux actions const increment = () => { return { type: "INCREMENT" }; }; // Redux reducer const counterReducer = (state = { count: 0 }, action) => { switch (action.type) { case "INCREMENT": return { count: state.count + 1 }; default: return state; } }; // Redux store const store = createStore(counterReducer); // React component connected to Redux store const CounterComponent = () => { const count = useSelector((state) => state.count); const dispatch = useDispatch(); return ( <div> <p>Count: {count}</p> <button onClick={() => dispatch(increment())}>Increment</button> </div> ); };
In this example, actions, a reducer, and a Redux store are defined. The
CounterComponent
connects to the Redux store using theuseSelector
anduseDispatch
hooks.
Understanding state management in React is crucial for building scalable and maintainable applications. The Context API provides a way to share state between components without prop drilling. State lifting is a pattern that simplifies state management by lifting state up to a common ancestor. Redux is a powerful state management library that introduces a predictable state container for managing the state of the entire application.
Module 10: Routing in React:
React Router:
-
Introduction to React Router: React Router is a standard library for routing in React applications. It enables the navigation between different components while keeping the UI in sync with the URL.
Installation:
npm install react-router-dom
Example:
import { BrowserRouter as Router, Route, Link } from "react-router-dom"; const Home = () => <h2>Home</h2>; const About = () => <h2>About</h2>; const App = () => { return ( <Router> <div> <nav> <ul> <li> <Link to="/">Home</Link> </li> <li> <Link to="/about">About</Link> </li> </ul> </nav> <Route path="/" exact component={Home} /> <Route path="/about" component={About} /> </div> </Router> ); };
In this example, the
Link
component is used for navigation, and theRoute
component defines the content to render based on the URL.
Navigation and Route Parameters:
-
Navigation with
useHistory
Hook: TheuseHistory
hook from React Router provides a way to navigate programmatically.Example:
import { useHistory } from "react-router-dom"; const NavigationComponent = () => { const history = useHistory(); const handleClick = () => { history.push("/about"); }; return ( <div> <button onClick={handleClick}>Go to About</button> </div> ); };
In this example, clicking the button navigates to the '/about' route using the
history.push
method. -
Route Parameters: Route parameters are used to capture dynamic segments of the URL.
Example:
const UserProfile = ({ match }) => { const { username } = match.params; return <h2>User Profile: {username}</h2>; }; const App = () => { return ( <Router> <Route path="/user/:username" component={UserProfile} /> </Router> ); };
In this example, the ':username' part of the route becomes a parameter accessible through the
match.params
object.
Nested Routes:
-
Nested Routes with React Router: React Router supports nested routes, allowing components to be nested in a hierarchy.
Example:
const Dashboard = () => <h2>Dashboard</h2>; const Profile = () => <h2>Profile</h2>; const App = () => { return ( <Router> <Route path="/dashboard" component={Dashboard} /> <Route path="/profile" component={Profile} /> </Router> ); };
In this example, '/dashboard' and '/profile' are top-level routes, but they could have their nested routes by rendering additional
Route
components inside their components.
Routing is a crucial part of single-page applications, allowing users to navigate between different views or pages. React Router simplifies the process of handling routes in React applications, providing components like Link
and Route
for navigation and rendering content based on the URL. Navigation can be achieved both through user interaction (e.g., clicking a link) and programmatically using hooks like useHistory
. Route parameters enable dynamic content based on the URL, and nested routes provide a way to structure components hierarchically.
Module 11: Hooks:
useState:
-
Introduction to
useState
: TheuseState
hook is used in functional components to add state to the component.Example:
import React, { useState } from "react"; const Counter = () => { const [count, setCount] = useState(0); const increment = () => { setCount(count + 1); }; return ( <div> <p>Count: {count}</p> <button onClick={increment}>Increment</button> </div> ); };
In this example,
useState(0)
initializes the state variablecount
with an initial value of 0. ThesetCount
function is used to update the state.
useEffect:
-
Introduction to
useEffect
: TheuseEffect
hook is used for side effects in functional components, such as data fetching, subscriptions, or manually changing the DOM.Example:
import React, { useState, useEffect } from "react"; const DataFetchingComponent = () => { const [data, setData] = useState([]); useEffect(() => { // Perform data fetching or other side effects // Update state with the fetched data fetch("https://api.example.com/data") .then((response) => response.json()) .then((result) => setData(result)); }, []); // Empty dependency array means it runs once (like componentDidMount) return ( <div> <ul> {data.map((item) => ( <li key={item.id}>{item.name}</li> ))} </ul> </div> ); };
In this example, the
useEffect
hook is used to fetch data from an API when the component mounts.
useContext:
-
Introduction to
useContext
: TheuseContext
hook is used to consume values from a React context.Example:
import React, { useContext } from "react"; // Create a context const ThemeContext = React.createContext("light"); const ThemedComponent = () => { // Consume the context value const theme = useContext(ThemeContext); return <p>Current theme: {theme}</p>; };
In this example,
useContext
is used to consume the value from theThemeContext
.
Custom Hooks:
-
Creating a Custom Hook: Custom hooks are functions that use one or more built-in hooks to provide a specific piece of functionality. They can be reused across components.
Example:
import { useState, useEffect } from "react"; const useWindowWidth = () => { const [windowWidth, setWindowWidth] = useState(window.innerWidth); const handleResize = () => { setWindowWidth(window.innerWidth); }; useEffect(() => { window.addEventListener("resize", handleResize); return () => { window.removeEventListener("resize", handleResize); }; }, []); // Empty dependency array means it runs once (like componentDidMount) return windowWidth; }; const WindowWidthComponent = () => { const width = useWindowWidth(); return <p>Window Width: {width}</p>; };
In this example,
useWindowWidth
is a custom hook that tracks the window width. The hook is then used in theWindowWidthComponent
.
Hooks are a powerful feature introduced in React to handle state, side effects, and context in functional components. useState
allows adding state to functional components, useEffect
handles side effects, and useContext
consumes values from a context. Custom hooks provide a way to encapsulate and reuse complex functionality across components, promoting code reusability and maintainability.
Module 12: Higher-Order Components (HOC):
What are HOCs?
- Introduction to Higher-Order Components (HOC): Higher-Order Components (HOCs) are functions that take a component and return a new component with enhanced functionality. They are a way to reuse component logic, share code between components, and perform common tasks.
Creating and Using HOCs:
-
Creating an HOC: An HOC is a function that takes a component and returns a new component with added props or behavior.
Example:
import React from "react"; const withLogger = (WrappedComponent) => { class WithLogger extends React.Component { componentDidMount() { console.log(`Component ${WrappedComponent.name} mounted`); } render() { return <WrappedComponent {...this.props} />; } } return WithLogger; }; const MyComponent = () => <p>My Component</p>; const MyComponentWithLogger = withLogger(MyComponent);
In this example,
withLogger
is an HOC that logs when the component it wraps is mounted. -
Using an HOC: The enhanced component returned by the HOC can be used in place of the original component.
Example:
const App = () => { return ( <div> <MyComponentWithLogger /> </div> ); };
In this example,
MyComponentWithLogger
is used in theApp
component, and the log statement is triggered when it mounts.
HOCs provide a way to reuse and share component logic. They are especially useful for cross-cutting concerns such as logging, authentication, or data fetching. By creating an HOC, you can encapsulate common functionality and apply it to multiple components. The HOC pattern enhances component composability and promotes code reuse in React applications.
Module 13: Render Props:
Render Props Pattern:
- Introduction to Render Props:
The Render Props pattern is a technique in React where a component receives a function as a prop, often called
render
orchildren
, which it then calls to render its output. This pattern is used to share code and state between components.
Using Render Props for Component Composition:
-
Creating a Render Prop Component: A component with a render prop takes a function as a prop and calls it to render its content.
Example:
import React from "react"; class MouseTracker extends React.Component { state = { x: 0, y: 0 }; handleMouseMove = (event) => { this.setState({ x: event.clientX, y: event.clientY }); }; render() { return ( <div style={{ height: "100vh" }} onMouseMove={this.handleMouseMove} > {this.props.render(this.state)} </div> ); } }
In this example,
MouseTracker
tracks the mouse position and calls therender
prop function with the current mouse coordinates. -
Using Render Prop Component: To use the
MouseTracker
component, provide a function as therender
prop to define how the mouse position should be displayed.Example:
const App = () => { return ( <MouseTracker render={({ x, y }) => ( <p> Mouse position: {x}, {y} </p> )} /> ); };
In this example, the
App
component uses theMouseTracker
with a render prop function that displays the current mouse position.
The Render Props pattern is a flexible way to share functionality between components. It allows components to delegate the rendering logic to the consuming component, enabling more dynamic and reusable components. Render props are often used for cross-cutting concerns or behaviors that can vary across different contexts. This pattern enhances component composability and encourages a more flexible and composable component architecture.
Module 14: Testing in React:
Jest and Enzyme:
-
Introduction to Jest and Enzyme: Jest is a JavaScript testing framework commonly used for React applications. Enzyme is a testing utility for React that makes it easier to test React components.
Example:
npm install --save-dev jest enzyme enzyme-to-json enzyme-adapter-react-16
-
Setting Up Jest and Enzyme: Create a
setupTests.js
file in your project to configure Enzyme with Jest.setupTests.js
:import { configure } from "enzyme"; import Adapter from "enzyme-adapter-react-16"; configure({ adapter: new Adapter() });
Include this setup file in your
package.json
or Jest configuration.
Testing Components and Hooks:
-
Component Testing with Enzyme: Use Enzyme's
shallow
ormount
functions to render components for testing.Example:
import { shallow } from "enzyme"; import MyComponent from "./MyComponent"; describe("MyComponent", () => { it("renders correctly", () => { const wrapper = shallow(<MyComponent />); expect(wrapper).toMatchSnapshot(); }); it("handles click event", () => { const mockClick = jest.fn(); const wrapper = shallow(<MyComponent onClick={mockClick} />); wrapper.find("button").simulate("click"); expect(mockClick).toHaveBeenCalled(); }); });
-
Hook Testing with Jest: Test React hooks using Jest's testing utilities.
Example:
import { render, fireEvent } from "@testing-library/react"; import useCounter from "./useCounter"; describe("useCounter", () => { it("increments counter on button click", () => { const { getByText } = render(<TestComponent />); fireEvent.click(getByText("Increment")); expect(getByText("Count: 1")).toBeInTheDocument(); }); });
In this example, the
useCounter
hook is tested by rendering a component that uses the hook and asserting the behavior.
Snapshot Testing:
-
Introduction to Snapshot Testing: Snapshot testing is a feature of Jest that captures the rendered output of a component and saves it as a snapshot. Subsequent test runs compare the rendered output to the saved snapshot.
Example:
import renderer from "react-test-renderer"; import MyComponent from "./MyComponent"; test("MyComponent snapshot", () => { const tree = renderer.create(<MyComponent />).toJSON(); expect(tree).toMatchSnapshot(); });
In this example, the first run of the test will generate a snapshot. Subsequent runs will compare the rendered output to the saved snapshot.
Testing is a crucial aspect of software development to ensure the correctness and reliability of your application. Jest and Enzyme are widely used tools for testing React applications. Enzyme provides utility functions to render components and interact with them in tests. Jest's snapshot testing allows you to capture the rendered output and easily identify changes. Testing components involves writing test cases to verify the rendering, behavior, and interactions of your components. Testing hooks can be done by rendering a component that uses the hook and asserting the expected behavior. Overall, testing is essential for maintaining a healthy codebase and preventing regressions.
Module 15: Styling in React:
CSS-in-JS Libraries (e.g., styled-components):
-
Introduction to CSS-in-JS Libraries: CSS-in-JS is an approach to styling in React where styles are defined directly within JavaScript files. It allows for scoped styles, dynamic theming, and better encapsulation.
-
Using
styled-components
:styled-components
is a popular CSS-in-JS library that allows you to write actual CSS code within your JavaScript components.Installation:
npm install styled-components
Example:
import styled from "styled-components"; const Button = styled.button` background-color: ${(props) => (props.primary ? "blue" : "white")}; color: ${(props) => (props.primary ? "white" : "black")}; padding: 10px 20px; border: 2px solid blue; `; const App = () => { return ( <div> <Button primary>Primary Button</Button> <Button>Secondary Button</Button> </div> ); };
In this example, the
Button
component is created usingstyled-components
. Theprimary
prop is used to conditionally apply styles.
CSS Modules:
-
Introduction to CSS Modules: CSS Modules are a way to locally scope your CSS in a modular fashion. It helps in avoiding global style conflicts and allows you to write modular stylesheets.
-
Using CSS Modules: With CSS Modules, you create a separate CSS file for each component and import the styles as an object in your JavaScript file.
Example:
// styles.module.css .button { background-color: white; color: black; padding: 10px 20px; border: 2px solid blue; } // ButtonComponent.jsx import React from 'react'; import styles from './styles.module.css'; const Button = () => { return ( <button className={styles.button}> Click me </button> ); };
In this example, the styles are defined in a separate CSS file (
styles.module.css
), and the styles are imported as an object in the JavaScript file.
Theming in React:
-
Theming with
styled-components
:styled-components
makes theming easy by allowing you to define themes and dynamically apply them to components.Example:
import { ThemeProvider } from "styled-components"; const theme = { primary: "blue", secondary: "green", }; const ThemedComponent = styled.div` background-color: ${(props) => props.theme.primary}; color: white; padding: 10px; `; const App = () => { return ( <ThemeProvider theme={theme}> <ThemedComponent>Themed Content</ThemedComponent> </ThemeProvider> ); };
In this example, the
ThemeProvider
is used to wrap components that need access to the theme. TheThemedComponent
uses thetheme
prop to apply styles.
Styling in React can be approached in various ways, and the choice depends on project requirements and personal preferences. CSS-in-JS libraries like styled-components
provide a convenient way to write and manage styles directly in your JavaScript components. CSS Modules offer a modular and locally scoped approach to styling, preventing global style conflicts. Theming in React, especially with styled-components
, allows you to define themes and dynamically apply them to components, providing a consistent and customizable design system. Each styling method has its advantages, and the choice depends on factors such as project size, team preferences, and styling requirements.
Module 16: Server Communication:
Fetch API:
-
Introduction to Fetch API: The Fetch API is a modern JavaScript API for making HTTP requests. It provides a simpler and more powerful alternative to traditional XMLHttpRequest.
-
Using Fetch in React: In React, you can use the Fetch API to make asynchronous requests to a server. The
fetch
function returns a Promise that resolves to the Response to that request.Example:
import React, { useEffect, useState } from "react"; const DataFetchingComponent = () => { const [data, setData] = useState([]); useEffect(() => { const fetchData = async () => { try { const response = await fetch( "https://api.example.com/data" ); const result = await response.json(); setData(result); } catch (error) { console.error("Error fetching data:", error); } }; fetchData(); }, []); return ( <div> <ul> {data.map((item) => ( <li key={item.id}>{item.name}</li> ))} </ul> </div> ); };
In this example, the
DataFetchingComponent
uses the Fetch API to fetch data from an API when the component mounts.
Axios:
-
Introduction to Axios: Axios is a popular JavaScript library for making HTTP requests. It is commonly used with React for its simplicity and additional features.
-
Using Axios in React: To use Axios in React, you need to install it and then use it to make asynchronous requests.
Installation:
npm install axios
Example:
import React, { useEffect, useState } from "react"; import axios from "axios"; const DataFetchingComponent = () => { const [data, setData] = useState([]); useEffect(() => { const fetchData = async () => { try { const response = await axios.get( "https://api.example.com/data" ); setData(response.data); } catch (error) { console.error("Error fetching data:", error); } }; fetchData(); }, []); return ( <div> <ul> {data.map((item) => ( <li key={item.id}>{item.name}</li> ))} </ul> </div> ); };
In this example, the
DataFetchingComponent
uses Axios to fetch data from the same API.
Asynchronous Programming in React:
-
Asynchronous Programming with
async/await
: Asynchronous programming is crucial when dealing with server communication in React. Theasync/await
syntax simplifies working with asynchronous code.Example:
import React, { useEffect, useState } from "react"; import axios from "axios"; const DataFetchingComponent = () => { const [data, setData] = useState([]); const [loading, setLoading] = useState(true); useEffect(() => { const fetchData = async () => { try { const response = await axios.get( "https://api.example.com/data" ); setData(response.data); } catch (error) { console.error("Error fetching data:", error); } finally { setLoading(false); } }; fetchData(); }, []); if (loading) { return <p>Loading...</p>; } return ( <div> <ul> {data.map((item) => ( <li key={item.id}>{item.name}</li> ))} </ul> </div> ); };
In this example, the
async/await
syntax is used to make the asynchronous Axios request. Theloading
state is used to display a loading message while the data is being fetched.
Server communication is a common requirement in React applications, especially for fetching data from APIs. The Fetch API and Axios are two popular options for making HTTP requests. The Fetch API is a built-in browser feature, while Axios is a third-party library with additional features. Both methods allow for asynchronous programming using async/await
, simplifying the handling of asynchronous code. When working with server communication in React components, it's essential to manage loading states and handle errors gracefully to provide a good user experience.
Module 17: Optimizing Performance:
Memoization:
-
Introduction to Memoization: Memoization is an optimization technique where the results of expensive function calls are cached so that subsequent calls with the same inputs can return the cached result instead of recalculating.
-
Using Memoization in React: In React, memoization can be applied to functional components using the
useMemo
hook or to class components using theReact.memo
higher-order component.Example (useMemo):
import React, { useMemo } from "react"; const ExpensiveComponent = ({ data }) => { const processedData = useMemo(() => { // Expensive data processing logic return data.map((item) => item * 2); }, [data]); return ( <div> {processedData.map((item) => ( <p key={item}>{item}</p> ))} </div> ); };
In this example, the
useMemo
hook is used to memoize the result of an expensive data processing operation based on thedata
prop.
PureComponent and React.memo:
-
Introduction to PureComponent and React.memo:
PureComponent
is a base class for class components in React that implements a shallow prop and state comparison to prevent unnecessary renders.React.memo
is the functional component equivalent. -
Using PureComponent: When a component extends
PureComponent
, it automatically performs a shallow comparison of props and state before deciding to re-render.Example:
import React, { PureComponent } from "react"; class PureExample extends PureComponent { render() { console.log("Rendered!"); return <p>PureComponent Example</p>; } }
In this example, the
PureExample
component will only re-render if the props or state values change. -
Using React.memo:
React.memo
can be used to memoize the result of a functional component, preventing re-renders if the props haven't changed.Example:
import React, { memo } from "react"; const MemoizedComponent = memo(({ data }) => { console.log("Rendered!"); return <p>Memoized Component</p>; });
In this example, the
MemoizedComponent
will only re-render if thedata
prop changes.
Performance Tools:
-
React DevTools and Profiler: React DevTools is a browser extension that allows you to inspect and debug React components. The Profiler tool can be used to identify performance bottlenecks.
Example:
-
React.StrictMode:
React.StrictMode
is a wrapper component that helps catch common mistakes and avoid potential issues early in development. It highlights potential problems in the console during development.Example:
import React from "react"; const App = () => { return <React.StrictMode>{/* Your app components */}</React.StrictMode>; };
In this example, the entire application is wrapped in
React.StrictMode
to enable additional checks and warnings.
Optimizing performance in React is crucial for creating smooth and responsive user interfaces. Memoization helps prevent unnecessary calculations by caching the results of expensive operations. PureComponent
and React.memo
aid in avoiding unnecessary renders by performing shallow comparisons of props and state. React DevTools and the Profiler tool assist in identifying performance issues during development, allowing for efficient optimization. Additionally, using React.StrictMode
helps catch potential problems early in the development process, ensuring that the application follows best practices for performance optimization.
Module 18: Progressive Web Apps (PWA):
Introduction to PWA:
- What is a Progressive Web App (PWA): A Progressive Web App (PWA) is a type of application software delivered through the web, built using common web technologies such as HTML, CSS, and JavaScript. PWAs are designed to work on any platform with a consistent user experience.
Service Workers:
-
Introduction to Service Workers: Service workers are scripts that run in the background, separate from a web page, and enable features such as push notifications, background sync, and caching.
-
Registering a Service Worker: To use a service worker in a React app, you need to register it. This is typically done in the main JavaScript file or a service worker registration file.
Example (index.js or serviceWorkerRegistration.js):
if ("serviceWorker" in navigator) { navigator.serviceWorker .register("/service-worker.js") .then((registration) => { console.log( "Service Worker registered with scope:", registration.scope ); }) .catch((error) => { console.error("Error registering Service Worker:", error); }); }
In this example, the service worker is registered with the file name
service-worker.js
. -
Service Worker File (service-worker.js): The service worker script is responsible for handling events and caching assets.
Example (service-worker.js):
self.addEventListener("install", (event) => { event.waitUntil( caches.open("my-cache").then((cache) => { return cache.addAll([ "/", "/index.html", "/app.js", // Add more assets to cache ]); }) ); }); self.addEventListener("fetch", (event) => { event.respondWith( caches.match(event.request).then((response) => { return response || fetch(event.request); }) ); });
In this example, the service worker installs and caches assets during the installation phase. During the fetch event, it serves cached assets if available.
Offline Capabilities:
-
Caching for Offline Access: One of the key features of PWAs is the ability to work offline. By caching assets, a PWA can still function even when the user is not connected to the internet.
-
Using Cached Assets: When a user visits a PWA, the service worker intercepts network requests and checks if the requested asset is in the cache. If it is, the cached version is served, providing a seamless offline experience.
Example (service-worker.js):
self.addEventListener("fetch", (event) => { event.respondWith( caches.match(event.request).then((response) => { return response || fetch(event.request); }) ); });
In this example, during the fetch event, the service worker checks the cache for the requested asset. If found, it serves the cached version; otherwise, it fetches the asset from the network.
Progressive Web Apps leverage service workers to provide enhanced capabilities, including offline access. Service workers are scripts that run in the background, enabling features like caching, push notifications, and background sync. To create a PWA with React, you register a service worker and implement caching strategies to store and retrieve assets, ensuring a reliable user experience even in offline mode. The use of service workers is a key aspect of PWAs, enabling developers to build web applications that behave more like native apps and work seamlessly across different devices and network conditions.
Module 19: Advanced React Patterns:
Render Props:
-
Render Props Pattern: The Render Props pattern is a technique in React where a component receives a function as a prop, often called
render
orchildren
, which it then calls to render its output. This pattern is used for sharing code and state between components.Example:
import React, { useState } from "react"; const MouseTracker = ({ render }) => { const [position, setPosition] = useState({ x: 0, y: 0 }); const handleMouseMove = (event) => { setPosition({ x: event.clientX, y: event.clientY }); }; return ( <div style={{ height: "100vh" }} onMouseMove={handleMouseMove}> {render(position)} </div> ); }; const App = () => { return ( <MouseTracker render={({ x, y }) => ( <p> Mouse position: {x}, {y} </p> )} /> ); };
In this example, the
MouseTracker
component uses the Render Props pattern to pass the mouse position to the rendering function.
Hooks Patterns (useReducer, useContext, etc.):
-
Hooks Patterns: React hooks, such as
useReducer
anduseContext
, provide powerful patterns for managing state and sharing logic between components.Example (useReducer):
import React, { useReducer } from "react"; const initialState = { count: 0 }; const reducer = (state, action) => { switch (action.type) { case "increment": return { count: state.count + 1 }; case "decrement": return { count: state.count - 1 }; default: return state; } }; const Counter = () => { const [state, dispatch] = useReducer(reducer, initialState); return ( <div> <p>Count: {state.count}</p> <button onClick={() => dispatch({ type: "increment" })}> Increment </button> <button onClick={() => dispatch({ type: "decrement" })}> Decrement </button> </div> ); };
In this example, the
useReducer
hook is used to manage state in theCounter
component. -
Example (useContext):
import React, { useContext } from "react"; const ThemeContext = React.createContext("light"); const ThemedComponent = () => { const theme = useContext(ThemeContext); return <p>Current Theme: {theme}</p>; }; const App = () => { return ( <ThemeContext.Provider value="dark"> <ThemedComponent /> </ThemeContext.Provider> ); };
In this example, the
useContext
hook is used to consume the theme value from theThemeContext
in theThemedComponent
.
Compound Components:
-
Compound Components: Compound Components is a pattern where a parent component manages the state and behavior, while child components are used to structure the UI.
Example:
import React, { useState } from "react"; const Accordion = ({ children }) => { const [openIndex, setOpenIndex] = useState(null); const handleToggle = (index) => { setOpenIndex((prevIndex) => (prevIndex === index ? null : index)); }; return React.Children.map(children, (child, index) => React.cloneElement(child, { isOpen: index === openIndex, onToggle: () => handleToggle(index), }) ); }; const AccordionItem = ({ isOpen, onToggle, title, children }) => { return ( <div> <div onClick={onToggle} style={{ cursor: "pointer" }}> <h3>{title}</h3> </div> {isOpen && <div>{children}</div>} </div> ); }; const App = () => { return ( <Accordion> <AccordionItem title="Section 1"> Content for Section 1 </AccordionItem> <AccordionItem title="Section 2"> Content for Section 2 </AccordionItem> <AccordionItem title="Section 3"> Content for Section 3 </AccordionItem> </Accordion> ); };
In this example, the
Accordion
component manages the state, andAccordionItem
components are used to structure the UI. TheAccordion
passes state and behavior down to theAccordionItem
components.
Advanced React patterns, such as Render Props, Hooks Patterns (e.g., useReducer
, useContext
), and Compound Components, provide powerful tools for building flexible and maintainable React applications. Render Props allow for the dynamic composition of components, Hooks Patterns enable state management and context sharing, and Compound Components enable the creation of reusable and encapsulated components. These patterns contribute to code organization, reusability, and maintainability in larger React projects.
Module 20: React Best Practices:
Code Splitting:
-
Code Splitting Best Practices: Code splitting is a technique used to improve the performance of a React application by splitting the bundle into smaller chunks. This helps in loading only the necessary code for a particular view or feature when it is required.
Example (React.lazy and Suspense):
import React, { lazy, Suspense } from "react"; const LazyComponent = lazy(() => import("./LazyComponent")); const App = () => { return ( <div> <Suspense fallback={<div>Loading...</div>}> <LazyComponent /> </Suspense> </div> ); };
In this example,
React.lazy
is used to create a dynamic import of theLazyComponent
. TheSuspense
component is used to show a fallback UI while the component is being loaded.
Error Boundaries:
-
Error Boundary Best Practices: Error boundaries are components that catch JavaScript errors anywhere in their child component tree and log those errors or display a fallback UI instead of crashing the component tree.
Example:
import React, { Component } from "react"; class ErrorBoundary extends Component { state = { hasError: false, error: null }; static getDerivedStateFromError(error) { return { hasError: true, error }; } componentDidCatch(error, errorInfo) { // Log the error to an error reporting service logErrorToService(error, errorInfo); } render() { if (this.state.hasError) { return <p>Something went wrong.</p>; } return this.props.children; } } const App = () => { return ( <ErrorBoundary>{/* Your application components */}</ErrorBoundary> ); };
In this example, the
ErrorBoundary
component is used to catch errors in its child component tree. ThegetDerivedStateFromError
andcomponentDidCatch
lifecycle methods are utilized to handle errors.
Accessibility in React:
-
Accessibility Best Practices: Accessibility (a11y) is a crucial aspect of web development, ensuring that applications are usable by people with disabilities. React provides features and best practices to improve accessibility.
Example (Accessible Button):
import React from "react"; const AccessibleButton = ({ onClick, label }) => { return ( <button onClick={onClick} aria-label={label}> {label} </button> ); };
In this example, the
AccessibleButton
component includes anaria-label
attribute to provide an accessible label for screen readers.
React best practices include Code Splitting to optimize the loading performance of applications by dynamically importing components only when needed. Error Boundaries help in gracefully handling errors in the component tree, preventing the entire application from crashing. Accessibility is emphasized to ensure that React applications are usable by a diverse audience, with practices such as providing accessible labels and ensuring a good user experience for people with disabilities. Adopting these best practices contributes to the overall maintainability, performance, and user experience of React applications.
Module 21: Real-world Project:
Building a Complete React Application:
-
Overview: Building a complete React application involves integrating various concepts and features discussed throughout the React crash course. It includes creating multiple components, managing state and props, handling user interactions, and structuring the application for scalability.
-
Example (Task Manager App): Suppose we're building a simple Task Manager application. Here's a simplified code snippet illustrating the structure:
// App.js import React, { useState } from "react"; import TaskList from "./TaskList"; import AddTaskForm from "./AddTaskForm"; const App = () => { const [tasks, setTasks] = useState([]); const addTask = (task) => { setTasks([...tasks, task]); }; return ( <div> <h1>Task Manager</h1> <AddTaskForm onAddTask={addTask} /> <TaskList tasks={tasks} /> </div> ); }; export default App;
In this example,
App
is the main component that manages the state of tasks and renders child components,AddTaskForm
for adding tasks, andTaskList
for displaying tasks.
Integrating with Backend Services:
-
Overview: Real-world applications often require integration with backend services to store and retrieve data. This involves making HTTP requests to APIs, handling responses, and updating the application state accordingly.
-
Example (Task Manager with Backend Integration): Suppose we have a backend API for managing tasks. We can use the
fetch
API or a library like Axios to interact with the backend.// App.js import React, { useState, useEffect } from "react"; import TaskList from "./TaskList"; import AddTaskForm from "./AddTaskForm"; const App = () => { const [tasks, setTasks] = useState([]); useEffect(() => { // Fetch tasks from backend API fetch("https://api.example.com/tasks") .then((response) => response.json()) .then((data) => setTasks(data)) .catch((error) => console.error("Error fetching tasks:", error) ); }, []); const addTask = (task) => { // Post new task to backend API fetch("https://api.example.com/tasks", { method: "POST", headers: { "Content-Type": "application/json", }, body: JSON.stringify(task), }) .then((response) => response.json()) .then((newTask) => setTasks([...tasks, newTask])) .catch((error) => console.error("Error adding task:", error)); }; return ( <div> <h1>Task Manager</h1> <AddTaskForm onAddTask={addTask} /> <TaskList tasks={tasks} /> </div> ); }; export default App;
In this example,
useEffect
is used to fetch tasks from the backend API when the component mounts. TheaddTask
function makes a POST request to add a new task.
Deployment Strategies:
-
Overview: Deploying a React application involves making it accessible to users on the internet. Various deployment strategies exist, including static hosting, serverless deployments, and traditional server deployments.
-
Example (Deploying to Netlify): Netlify is a popular platform for deploying static sites and serverless functions. Assuming you have a Netlify account and the application is in a Git repository, deploying to Netlify is as simple as connecting the repository and configuring build settings.
- Connect repository to Netlify.
- Set build settings (e.g., build command, publish directory).
- Deploy the application.
Netlify automatically builds and deploys the application, providing a URL for access.
Conclusion:
Building a real-world React project involves combining the concepts learned in the crash course to create a complete and functional application. Integrating with backend services allows the application to persist data, and choosing an appropriate deployment strategy makes it accessible to users. This module serves as a practical guide to apply React knowledge in real-world scenarios, emphasizing the importance of structuring components, managing state, and connecting with external services for a comprehensive development experience.
Module 22: Interview Preparation:
Common React Interview Questions:
-
Overview: React interviews often cover fundamental concepts, best practices, and the ability to solve problems. Common questions may revolve around React components, state management, lifecycle methods, and performance optimization.
-
Example Questions:
- Explain the difference between functional components and class components in React.
- What is JSX, and how does it differ from HTML?
- How does state differ from props in React?
- What are controlled and uncontrolled components in React forms?
- Explain the React component lifecycle methods.
Problem-Solving with React:
-
Overview: Problem-solving skills are crucial for React interviews. Interviewers may ask candidates to solve coding challenges or architectural problems related to React applications.
-
Example Problem: Problem Statement: Implement a simple counter component in React that increments or decrements a value based on user actions.
// Counter.js import React, { useState } from "react"; const Counter = () => { const [count, setCount] = useState(0); const increment = () => setCount(count + 1); const decrement = () => setCount(count - 1); return ( <div> <p>Count: {count}</p> <button onClick={increment}>Increment</button> <button onClick={decrement}>Decrement</button> </div> ); }; export default Counter;
Explanation: In this example, the
Counter
component uses theuseState
hook to manage the state of the count. Two buttons trigger theincrement
anddecrement
functions, updating the count and re-rendering the component.
System Design Considerations:
-
Overview: System design questions in React interviews may focus on how candidates approach building scalable and maintainable applications. Topics may include component structure, state management, and optimizing performance.
-
Example Considerations:
-
Component Hierarchy:
- How would you structure components for a complex user interface?
- What factors influence your decision on component hierarchy?
-
State Management:
- How do you decide whether to use local component state or global state management (e.g., Redux)?
- Explain the concept of lifting state up in React.
-
Performance Optimization:
- What strategies would you employ to optimize the performance of a React application?
- How does code splitting contribute to performance optimization?
-
Conclusion:
Interview preparation for React involves a combination of understanding common interview questions, demonstrating problem-solving skills through coding challenges, and considering system design principles. Candidates should be well-versed in React concepts, be able to apply them to real-world scenarios, and articulate their design decisions. The provided examples showcase how candidates can approach answering questions and solving problems during a React interview, emphasizing the importance of practical application and a deep understanding of React fundamentals.
Summary
1. Introduction to React:
- What is React.js?
- Why use React.js?
- Virtual DOM concept
- JSX syntax
2. Setting Up Your Development Environment:
- Node.js and npm installation
- Create React App
- Understanding the project structure
3. Components and Props:
- Functional components
- Class components
- Props and PropTypes
- State and setState
4. JSX (JavaScript XML):
- Understanding JSX syntax
- JSX expressions
- Conditional rendering in JSX
5. Handling Events:
- Event handling in React
- Binding event handlers
- Using arrow functions for event handlers
6. Lists and Keys:
- Rendering lists in React
- Keys and their importance
- Dynamic lists
7. Forms in React:
- Controlled components
- Form submission and handling
- Form validation
8. Component Lifecycle:
- Mounting, updating, and unmounting phases
- Lifecycle methods
- useEffect hook
9. State Management:
- Context API
- State lifting
- Redux (Introduction)
10. Routing in React:
- React Router
- Navigation and Route parameters
- Nested routes
11. Hooks:
- useState
- useEffect
- useContext
- Custom hooks
12. Higher-Order Components (HOC):
- What are HOCs?
- Creating and using HOCs
13. Render Props:
- Render props pattern
- Using render props for component composition
14. Testing in React:
- Jest and Enzyme
- Testing components and hooks
- Snapshot testing
15. Styling in React:
- CSS-in-JS libraries (e.g., styled-components)
- CSS modules
- Theming in React
16. Server Communication:
- Fetch API
- Axios
- Asynchronous programming in React
17. Optimizing Performance:
- Memoization
- PureComponent and React.memo
- Performance tools
18. Progressive Web Apps (PWA):
- Introduction to PWA
- Service workers
- Offline capabilities
19. Advanced React Patterns:
- Render props
- Hooks patterns (useReducer, useContext, etc.)
- Compound components
20. React Best Practices:
- Code splitting
- Error boundaries
- Accessibility in React
21. Real-world Project:
- Building a complete React application
- Integrating with backend services
- Deployment strategies
22. Interview Preparation:
- Common React interview questions
- Problem-solving with React
- System design considerations
This list covers a wide range of React.js topics, from the basics to advanced concepts. Remember that practical implementation and building projects are crucial for reinforcing your understanding of these concepts. Additionally, staying updated with the latest React.js features and best practices is essential in the ever-evolving field of web development.
React.js Crash Course
What is React.js?
React.js is a JavaScript library for building user interfaces. It is maintained by Facebook and a community of individual developers and companies. React can be used as a base in the development of single-page or mobile applications.
Why React.js?
React.js is a JavaScript library for building user interfaces. It is maintained by Facebook and a community of individual developers and companies. React can be used as a base in the development of single-page or mobile applications.
What is JSX?
JSX is a syntax extension to JavaScript. It is similar to a template language, but it has full power of JavaScript. JSX gets compiled to React.createElement() calls which return plain JavaScript objects called “React elements”. React then uses these elements to construct the DOM.
What is Virtual DOM?
The virtual DOM (VDOM) is a programming concept where an ideal, or “virtual”, representation of a UI is kept in memory and synced with the “real” DOM by a library such as ReactDOM. This process is called reconciliation.
What is the difference between Real DOM and Virtual DOM?
Real DOM | Virtual DOM |
---|---|
It updates slow | It updates faster |
Can directly update HTML | Can't directly update HTML |
Creates a new DOM if element updates | Updates the JSX if element updates |
DOM manipulation is very expensive | DOM manipulation is very easy |
Too much of memory wastage | No memory wastage |
What is the difference between state and props?
State | Props |
---|---|
State is mutable. | Props are immutable. |
State is local to the component. | Props can be accessed by child components. |
State can be changed by the component itself using setState() method. | Props can't be changed by the component itself. |
State affects only the component in which it is declared. | Props can affect several components, but only in one direction. |
State can be initialized inside the component. | Props can't be initialized inside the component. |
State is an object. | Props can be any data type, including functions. |
State is managed within the component. | Props are managed outside the component. |
State is used to update the component. | Props are used to pass data and event handlers from one component to another. |
What is the difference between Class and Functional Components?
Class Components | Functional Components |
---|---|
Class components are ES6 classes that extend from React.Component. | Functional components are functions. |
Class components can have state. | Functional components can't have state. |
Class components can have refs. | Functional components can't have refs. |
Class components can have lifecycle hooks. | Functional components can't have lifecycle hooks. |
Class components can use shouldComponentUpdate() to improve performance. | Functional components can't use shouldComponentUpdate() method. |
Class component is more verbose and has steep learning curve. | Functional components are less verbose and easy to grasp. |
Class components can be stateful or stateless. | Functional components are stateless. |
Class components are more flexible. | Functional components are less flexible. |
Class components are more complicated to write. | Functional components are less complicated to write. |
Class components are faster than functional components as they have shouldUpdate. | Functional components are slower than class components as they don't have shouldUpdate. |
What is the difference between Controlled and Uncontrolled Components?
Controlled Components | Uncontrolled Components |
---|---|
In controlled components, form data is handled by a React component. | In uncontrolled components, form data is handled by the DOM itself. |
In controlled components, the state of the component is initialized and updated by the component itself. | In uncontrolled components, the state of the component is handled by the DOM itself. |
In controlled components, to update the state, setState() method is used. | In uncontrolled components, a ref is used to update the state. |
Controlled components provide better performance. | Uncontrolled components don't provide better performance. |
Controlled components use more code as you need to write an event handler for every way your data can change and pipe all of the input state through a React component. | Uncontrolled components use less code as you don't need to write an event handler for every way your data can change. |
What is the difference between Shallow Rendering and Full Rendering?
Shallow Rendering | Full Rendering |
---|---|
Shallow rendering tests components in isolation from the child components. | Full rendering tests components in the DOM, which requires DOM and a browser. |
Shallow rendering is faster as it does not require DOM. | Full rendering is slower as it requires DOM. |
Shallow rendering is less suitable for testing the behavior of the child components. | Full rendering is more suitable for testing the behavior of the child components. |
What is the difference between createElement and cloneElement?
createElement | cloneElement |
---|---|
It is used to create an element. | It is used to clone an element and pass it new props. |
It accepts three arguments: type, props, children. | It accepts three arguments: element, props, children. |
It returns a React element. | It returns a React element. |
It is a top-level API. | It is a low-level API. |
It is used when you write JSX. | It is used when you don't write JSX. |
It is used to create a new element from a given element. | It is used to return a copy of the element with the updated props. |
It is used to create a new element from a React class or a function component. | It is used to create a new element from a given element. |
It is used to create a new element from a React class or a function component. | It is used to create a new element from a React class or a function component. |
It is used to create a new element from a React class or a function component. | It is used to create a new element from a React class or a function component. |
Add a Comment
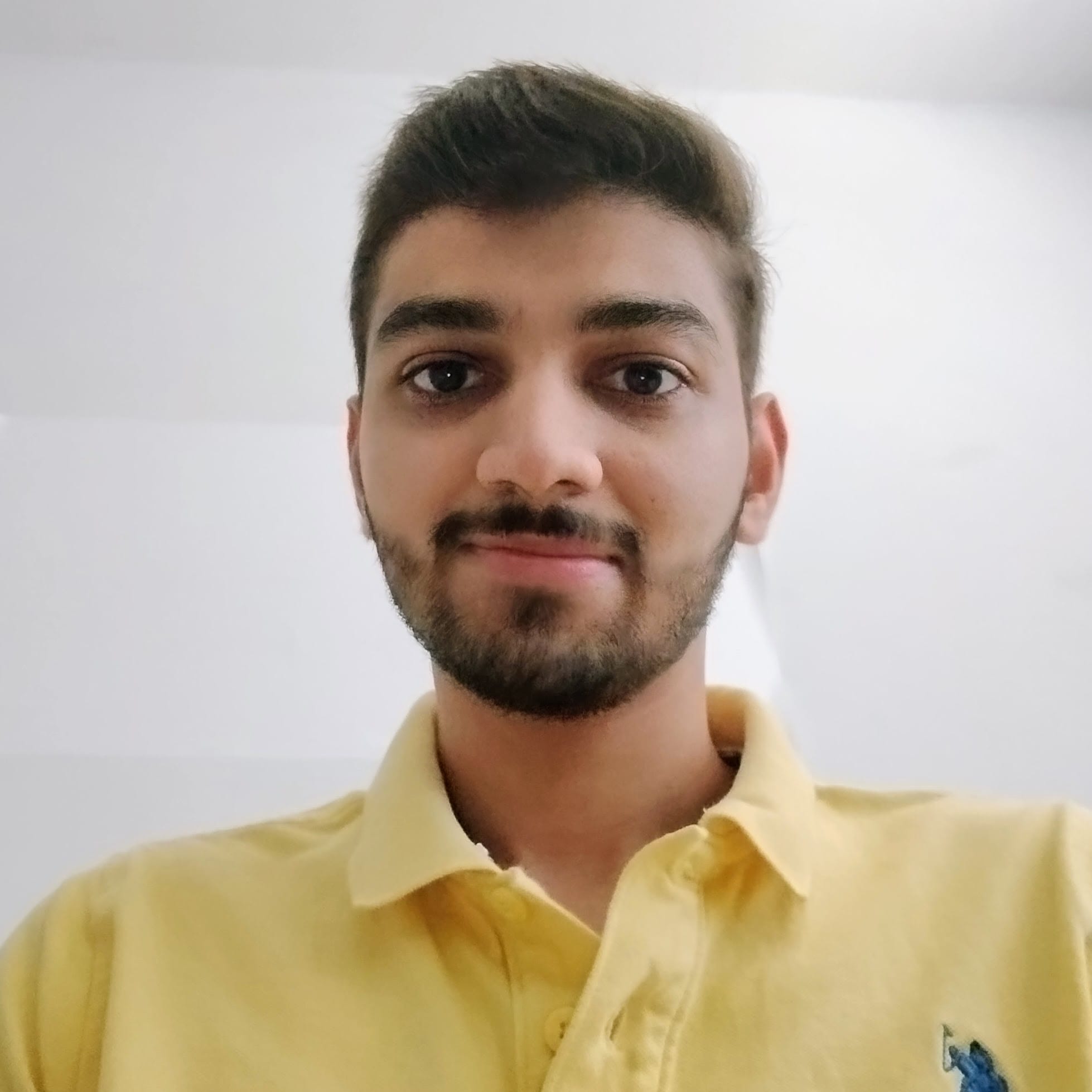
Archit Rathod
Software Developer and tech enthusiast. Passionate about web development and creating user-friendly experiences.